And Then You Were Gone
I probably overuse temp tables. I’m the first one to admit it. But often when I choose to use one, it’s a choice between:
- Spending a long time trying to business understand logic for a company I don’t work for
- Tinkering with query syntax and using really weird tricks and hints to get different plan shapes
- Waiting a long time to create or tweak indexes on huge tables (usually on “dev” hardware)
And you know what? If I had to work anywhere full time, and not balance performance tuning requests from a bunch of people, I just might spend more time on those things.
Don’t tell anyone, but sometimes if I’m really busy, I have no problem waiting a long time for an index change to go through, so I can look at something else for a while.
In general, temp tables offer a convenient way to focus on one problem part of the query, without the noise of a bazillion other joins and applys and subqueries and everything else going on.
They also offer a familiar paradigm to anyone looking at the work later, that doesn’t involve SELECT TOP (2147483647) UNION ALL SELECT TOP (1)
type syntax, which can really raise some eyebrows on unfamiliar faces.
A Recent Example
I’m using Stack Overflow in my query, but a recent client engagement had a problem query that looked about the same.
WITH top5 AS ( SELECT p.*, n = ROW_NUMBER() OVER ( PARTITION BY p.OwnerUserId ORDER BY p.Score DESC ) FROM dbo.Posts AS p WHERE p.OwnerUserId = 22656 AND p.PostTypeId = 1 ) SELECT u.DisplayName, t1.Title, t2.Title, t3.Title, t4.Title, t5.Title FROM dbo.Users AS u LEFT JOIN top5 AS t1 ON t1.OwnerUserId = u.Id AND t1.n = 1 LEFT JOIN top5 AS t2 ON t2.OwnerUserId = u.Id AND t2.n = 2 LEFT JOIN top5 AS t3 ON t3.OwnerUserId = u.Id AND t3.n = 3 LEFT JOIN top5 AS t4 ON t4.OwnerUserId = u.Id AND t4.n = 4 LEFT JOIN top5 AS t5 ON t5.OwnerUserId = u.Id AND t5.n = 5 WHERE t1.OwnerUserId IS NOT NULL;
The problems with this contain multitudes, but let’s let the query plan do the talking:
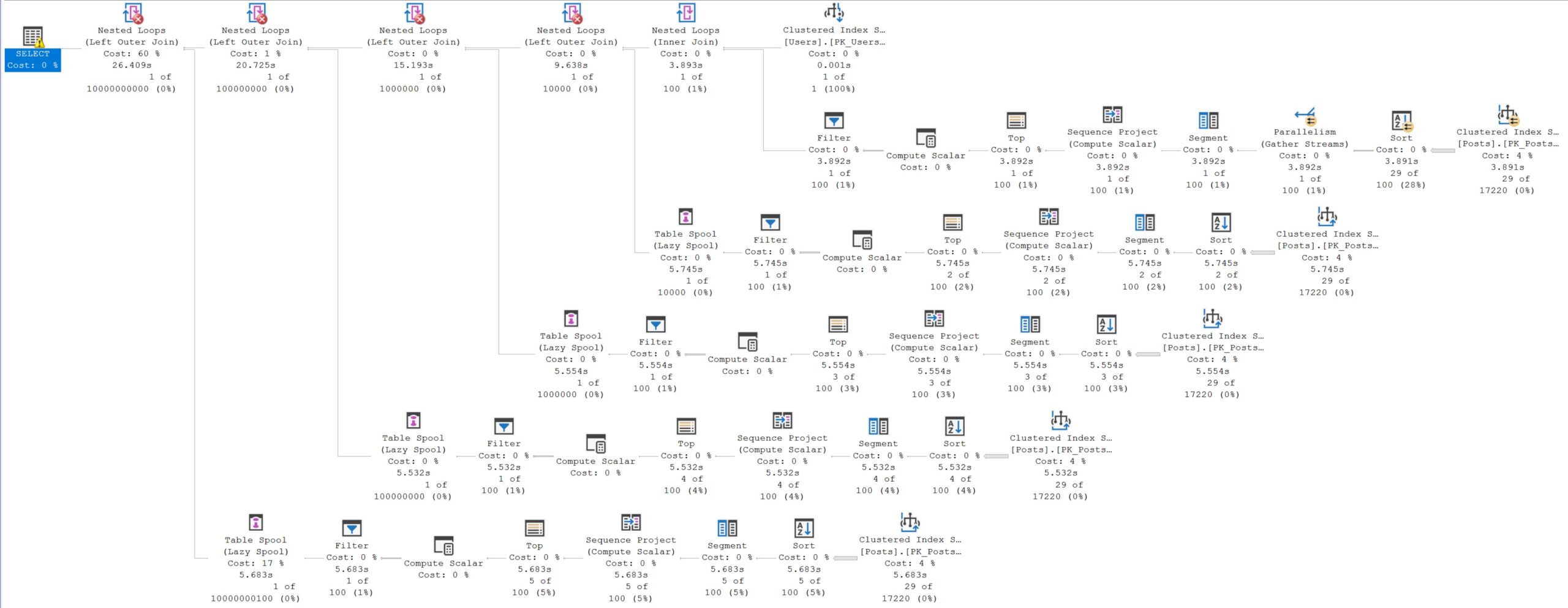
In the words of Drake: “Take Care”
If you’re the type of developer who insists that common table expressions are some sort of magic potion, I do hope that you this is your hangover.
Practical Magic
Temporary tables are the cure for common table expression hangovers, for the most part.
Let’s see how we do with one.
WITH top5 AS ( SELECT p.*, n = ROW_NUMBER() OVER ( PARTITION BY p.OwnerUserId ORDER BY p.Score DESC ) FROM dbo.Posts AS p WHERE p.OwnerUserId = 22656 AND p.PostTypeId = 1 ) SELECT t.OwnerUserId, t.Title, n INTO #top5 FROM top5 AS t WHERE t.n <= 5; SELECT u.DisplayName, t1.Title, t2.Title, t3.Title, t4.Title, t5.Title FROM dbo.Users AS u LEFT JOIN #top5 AS t1 ON t1.OwnerUserId = u.Id AND t1.n = 1 LEFT JOIN #top5 AS t2 ON t2.OwnerUserId = u.Id AND t2.n = 2 LEFT JOIN #top5 AS t3 ON t3.OwnerUserId = u.Id AND t3.n = 3 LEFT JOIN #top5 AS t4 ON t4.OwnerUserId = u.Id AND t4.n = 4 LEFT JOIN #top5 AS t5 ON t5.OwnerUserId = u.Id AND t5.n = 5 WHERE t1.OwnerUserId IS NOT NULL;
Here’s the new query plan:
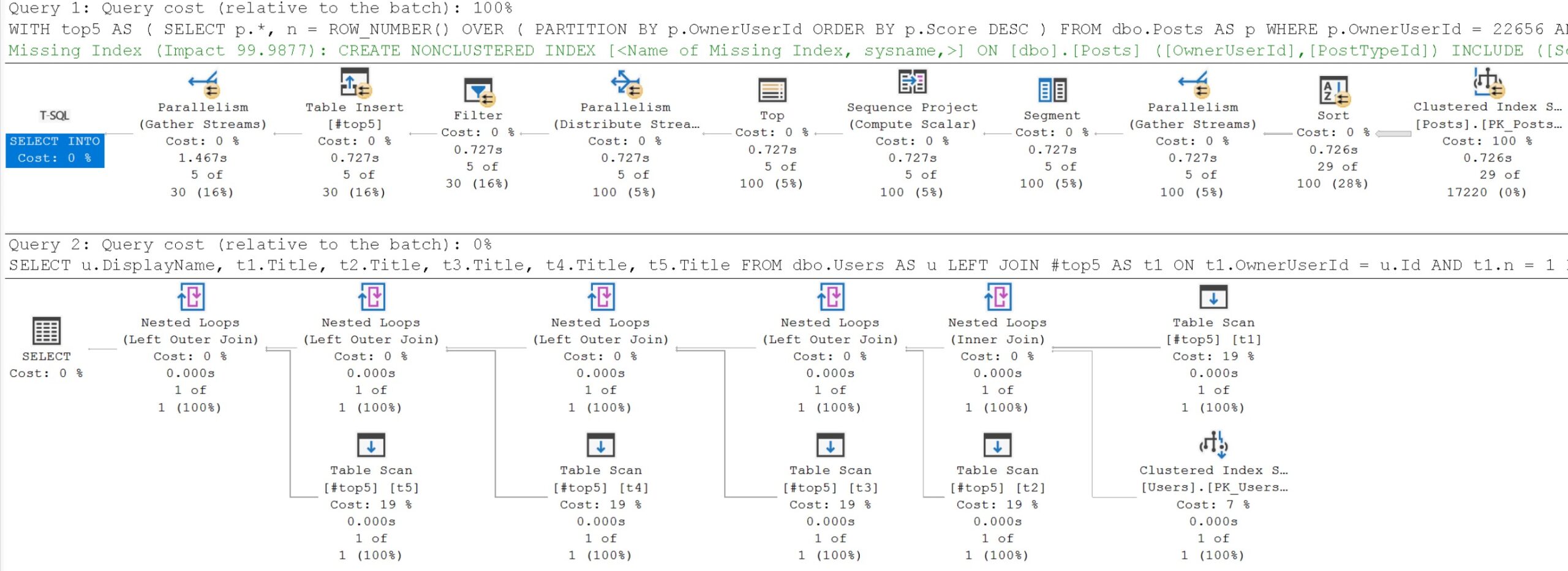
Without changing indexes, we get our query from 26 seconds to 1.5 seconds.
Really puts the “common” in “common table expressions”, doesn’t it?
Argue
While the “should I use common table expressions or temp tables?” question is largely a settled one for me, one thing that always comes up in seemingly chaotic practice is temp tables vs. table variables.
Table variables certainly have their place in the world, though often not without compromises or alternatives.
Many developers will use table variables for the purpose of passing them directly to another stored procedure. That’s fine and all, but you can just use #temp tables and reference them directly in another stored procedure, too.
Other times, which on gets used seems to be a question of moods and fancies of the developer currently working on something.
Some time ago I talked about when table variables are most useful in SQL Server practice.
And I stand by the majority of what’s discussed in here still. Hopefully you find it enlightening, even if you’ve already seen it.
Thanks for reading!
Going Further
If this is the kind of SQL Server stuff you love learning about, you’ll love my training. I’m offering a 75% discount to my blog readers if you click from here. I’m also available for consulting if you just don’t have time for that, and need to solve database performance problems quickly. You can also get a quick, low cost health check with no phone time required.
One thought on “The Art Of The SQL Server Stored Procedure: Temporary Objects”
Comments are closed.